Initializing a MongoDB Docker Image with Data
Prerequisites
- Docker installed on your machine
Get Mongo Running via Docker Compose
First, create an empty directory to house a few files related to our task:
mkdir docker-mongo-import-example
Then create a new docker-compose.yml file with the following contents:
# docker-compose.yml
services:
mongo:
image: mongo:latest
container_name: mongo
ports:
- "27017:27017"
environment:
MONGO_INITDB_ROOT_USERNAME: root
MONGO_INITDB_ROOT_PASSWORD: example
mongo-express:
image: mongo-express:latest
container_name: mongo-express
ports:
- "8081:8081"
environment:
ME_CONFIG_MONGODB_ADMINUSERNAME: root
ME_CONFIG_MONGODB_ADMINPASSWORD: example
ME_CONFIG_MONGODB_SERVER: mongo
ME_CONFIG_BASICAUTH: false
depends_on:
- mongo
This will create a Docker Compose stack with two containers: MongoDB and mongo-express, which can be used to visualize our database in the browser.
Run the Docker Compose stack with docker compose up --build
Once everything is up and running, you should be able to use mongo-express to view the contents of the MongoDB database. Try navigating to http://localhost:8081 in your browser, and you should see a page like:
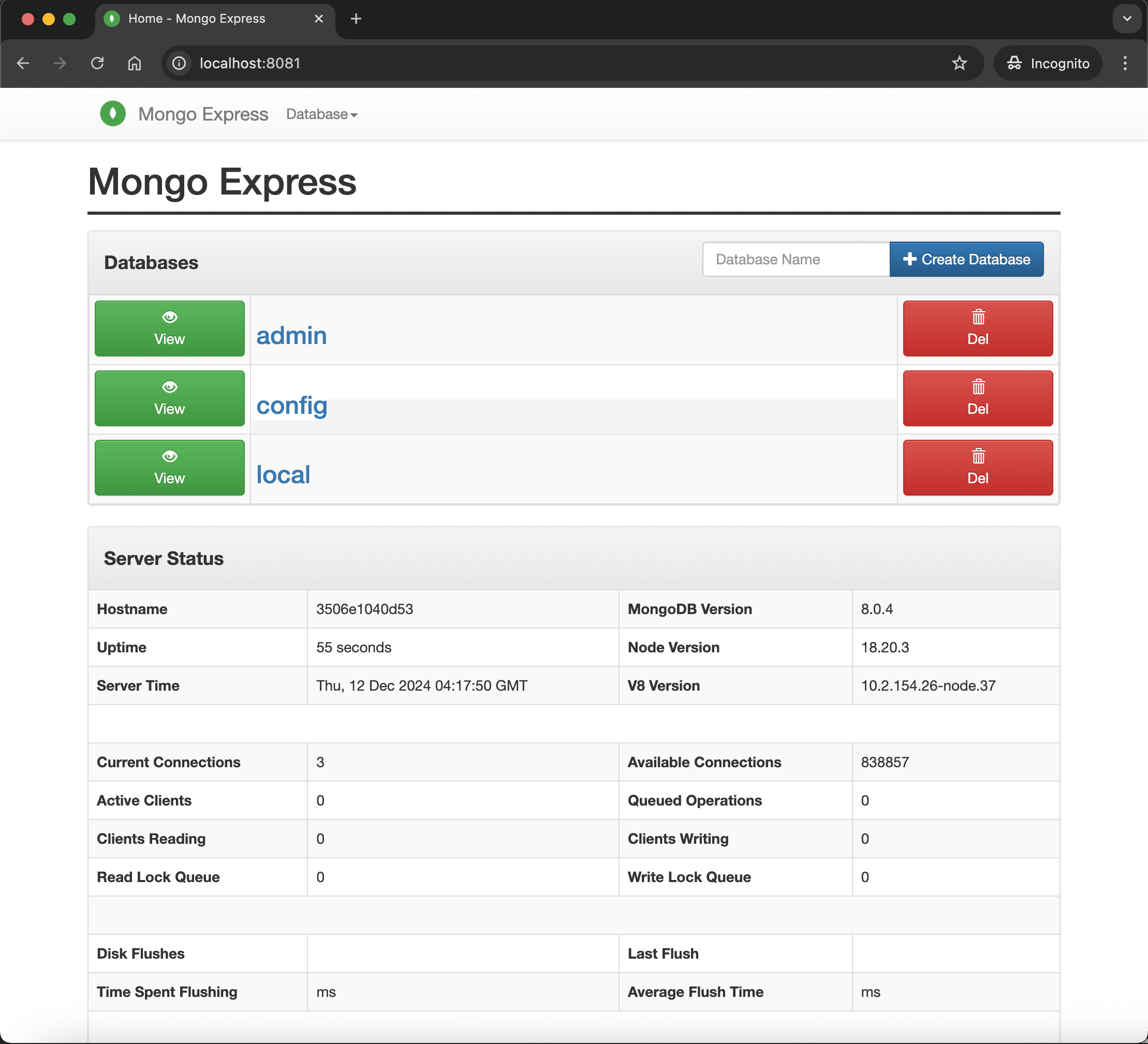
Try clicking around in the different databases that were created.
Not much to look at, huh? Let's fix that.
Download some test data
MongoDB has some links to sample data sets that can easily be imported into a MongoDB database: https://www.mongodb.com/resources/basics/databases/sample-database
For this example, I am using https://insideairbnb.com/get-the-data/. I chose a listings.csv.gz
file for a city at random.
Download, unzip, and move the resulting listings.csv
file to a new directory in our folder: import-data
.
Add an import script
The MongoDB Docker image will run any .js or .sh files present in the /docker-entrypoint-initdb.d
directory in the Docker container at startup. We will leverage this to help us easily import our sample dataset.
Add a new file in our project folder: init-files/load-data.sh
# init-files/load-data.sh
mongoimport --host 0.0.0.0 -c collection --type csv --file /import-data/listings.csv --headerline
This will use the mongoimport utility to connect to the MongoDB database (0.0.0.0 as the host since this is running inside the MongoDB Docker container).
Mount the volumes
With the sample data and import script in place, mount the volumes in the MongoDB Docker container so that MongoDB can utilize them during container startup.
# docker-compose.yml
services:
mongo:
image: mongo:latest
container_name: mongo
ports:
- "27017:27017"
environment:
MONGO_INITDB_ROOT_USERNAME: root
MONGO_INITDB_ROOT_PASSWORD: example
MONGO_INITDB_DATABASE: listings
# Add volumes section here
volumes:
# The following mount will be used for the import scripts
- ./init-files:/docker-entrypoint-initdb.d
# The following mount will be used for the import data
- ./import-data:/import-data
# ...
Now, with everything in place, run docker compose down --volumes
(since we already initialized our MongoDB database earlier, we must remove the volume that was created) and then docker compose up --build
.
Success! If everything worked properly, when you visit http://localhost:8081, you should see a new database, test
. If you enter the database, then the collection
, you should see data from your import file:
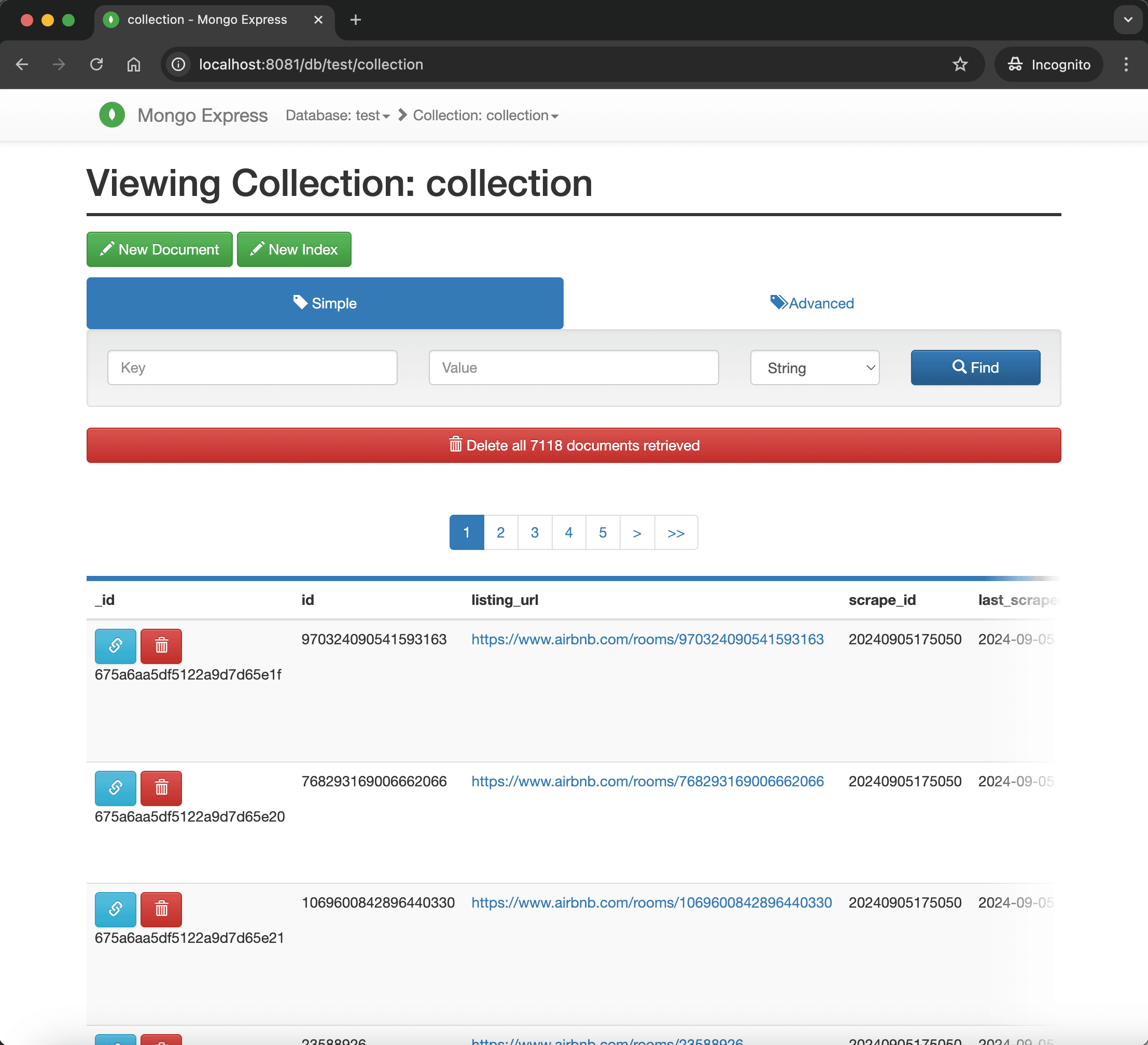
Importing JSON files
If you'd like to import a JSON file, you can use the following command:
mongoimport --collection collection --file /import-data/listings.json --jsonArray